2022. 1. 12. 13:00ㆍReact Native/Basic
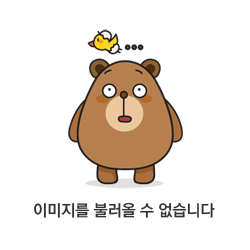
2022.01.11 - [React Native/Basic] - [React Native] React Native Component - 1. Intro
2022.01.12 - [React Native/Basic] - [React Native] React Native Component - 2. View, Text
2022.01.12 - [React Native/Basic] - [React Native] React Native Component - 3. Style
2022.01.12 - [React Native/Basic] - [React Native] React Native Component - 4. TouchEvent
2022.01.12 - [React Native/Basic] - [React Native] React Native Component - 5. Button
2022.01.12 - [React Native/Basic] - [React Native] React Native Component - 6. ScrollView
2022.01.12 - [React Native/Basic] - [React Native] React Native Component - 7. TextInput
2022.01.12 - [React Native/Basic] - [React Native] React Native Component - 9. Picker
2022.01.12 - [React Native/Basic] - [React Native] React Native Component - 10. Slider
2022.01.12 - [React Native/Basic] - [React Native] React Native Component - 11. ActivityIndicator
2022.01.12 - [React Native/Basic] - [React Native] React Native Component - 12. Image
2022.01.12 - [React Native/Basic] - [React Native] React Native Component - 13. Modal
4. TouchEvent
앱 상의 버튼을 누르면 액션이 발생한다. 버튼 뿐만 아니라 뷰나 텍스트에도 이벤트를 발생시킬 수 있다.
함수를 정의할 때 () 과 {} 의 차이.
exampleFunction = () => {
//return 되는 jsx 컴포넌트 없음.
}
exampleFunction = () => (
//jsx 컴포넌트 return
//jsx - javascript xml
)
jsx: javascript xml
html + javascript
예시
const example = <tag>hello world</tag>
header.js
/**
* Sample React Native App
* https://github.com/facebook/react-native
*
* @format
* @flow strict-local
*/
import React from 'react';
import {View, Text, StyleSheet} from 'react-native';
const Header = props => (
<View style={styles.header}>
<Text>{props.name}</Text>
</View>
);
const styles = StyleSheet.create({
header: {
backgroundColor: 'pink',
alignItems: 'center',
padding: 5,
width: '100%',
},
});
export default Header;
App.js
/**
* Sample React Native App
* https://github.com/facebook/react-native
*
* @format
* @flow strict-local
*/
import React, {Component} from 'react';
import {View, Text, StyleSheet} from 'react-native';
import Header from './src/header';
class App extends Component {
state = {
appName: 'My First App',
};
render() {
return (
<View style={styles.mainView}>
<Header name={this.state.appName} />
</View>
);
}
}
const styles = StyleSheet.create({
mainView: {
backgroundColor: 'white',
flex: 1,
paddingTop: 50,
alignItems: 'center',
justifyContent: 'center',
},
subView: {
backgroundColor: 'yellow',
marginBottom: 10,
},
anotherSubView: {
flex: 2,
backgroundColor: 'yellow',
marginBottom: 10,
width: '100%',
alignItems: 'center',
justifyContent: 'center',
},
mainText: {
fontSize: 20,
fontWeight: 'normal',
color: 'red',
padding: 20,
},
});
export default App;
TouchableOpacity - 뷰가 조금 투명해지면서 반응
https://reactnative.dev/docs/touchableopacity
/**
* Sample React Native App
* https://github.com/facebook/react-native
*
* @format
* @flow strict-local
*/
import React from 'react';
import {
View,
Text,
StyleSheet,
TouchableOpacity,
TouchableWithoutFeedback,
} from 'react-native';
const Header = props => (
<TouchableOpacity style={styles.header} onPress={() => alert('hello world')}>
<View>
<Text>{props.name}</Text>
</View>
</TouchableOpacity>
);
const styles = StyleSheet.create({
header: {
backgroundColor: 'pink',
alignItems: 'center',
padding: 5,
width: '100%',
},
});
export default Header;
TouchableWithoutFeedback - 뷰가 불투명. 뷰에 아무런 변화를 일으키지 않기 때문에 View에 style을 지정해줘야 한다.
https://reactnative.dev/docs/touchablewithoutfeedback#props
TouchableWithoutFeedback · React Native
If you're looking for a more extensive and future-proof way to handle touch-based input, check out the Pressable API.
reactnative.dev
/**
* Sample React Native App
* https://github.com/facebook/react-native
*
* @format
* @flow strict-local
*/
import React from 'react';
import {
View,
Text,
StyleSheet,
TouchableOpacity,
TouchableWithoutFeedback,
} from 'react-native';
const Header = props => (
<TouchableWithoutFeedback onPress={() => alert('hello world')}>
<View style={styles.header}>
<Text>{props.name}</Text>
</View>
</TouchableWithoutFeedback>
);
const styles = StyleSheet.create({
header: {
backgroundColor: 'pink',
alignItems: 'center',
padding: 5,
width: '100%',
},
});
export default Header;
Onpress - 눌렀을 때 반응
OnLongPress - 길게 눌렀을 때 반응
OnPressIn - 누르자마자 반응
OnpressOut - 눌렀던 것을 땔 때 반응
/**
* Sample React Native App
* https://github.com/facebook/react-native
*
* @format
* @flow strict-local
*/
import React from 'react';
import {
View,
Text,
StyleSheet,
TouchableOpacity,
TouchableWithoutFeedback,
} from 'react-native';
const Header = props => (
<TouchableOpacity
style={styles.header}
// onPress={() => alert('hello world')}
// onLongPress={() => alert('hello world')}
// onPressIn={() => alert('hello world')}
onPressOut={() => alert('hello world')}>
<View>
<Text>{props.name}</Text>
</View>
</TouchableOpacity>
);
const styles = StyleSheet.create({
header: {
backgroundColor: 'pink',
alignItems: 'center',
padding: 5,
width: '100%',
},
});
export default Header;
text에 이벤트 발생 예시
/**
* Sample React Native App
* https://github.com/facebook/react-native
*
* @format
* @flow strict-local
*/
import React, {Component} from 'react';
import {View, Text, StyleSheet} from 'react-native';
import Header from './src/header';
class App extends Component {
state = {
appName: 'My First App',
};
render() {
return (
<View style={styles.mainView}>
{/* <Header name={this.state.appName} /> */}
<Text style={styles.mainText} onPress={() => alert('text touch event')}>
Hello World
</Text>
</View>
);
}
}
const styles = StyleSheet.create({
mainView: {
backgroundColor: 'white',
flex: 1,
paddingTop: 50,
alignItems: 'center',
justifyContent: 'center',
},
subView: {
backgroundColor: 'yellow',
marginBottom: 10,
},
anotherSubView: {
flex: 2,
backgroundColor: 'yellow',
marginBottom: 10,
width: '100%',
alignItems: 'center',
justifyContent: 'center',
},
mainText: {
fontSize: 20,
fontWeight: 'normal',
color: 'red',
padding: 20,
},
});
export default App;
참고 자료
iOS/Android 앱 개발을 위한 실전 React Native - Basic - 인프런 | 강의
Mobile App Front-End 개발을 위한 React Native의 기초 지식 습득을 목표로 하고 있습니다. 진입장벽이 낮은 언어/API의 활용을 통해 비전문가도 쉽게 Native Mobile App을 개발할 수 있도록 제작된 강의입니다
www.inflearn.com
'React Native > Basic' 카테고리의 다른 글
[React Native] React Native Component - 6. ScrollView (0) | 2022.01.12 |
---|---|
[React Native] React Native Component - 5. Button (0) | 2022.01.12 |
[React Native] React Native Component - 3. Style (0) | 2022.01.12 |
[React Native] React Native Component - 2. View, Text (0) | 2022.01.12 |
[React Native] React Native Component - 1. Intro (0) | 2022.01.11 |